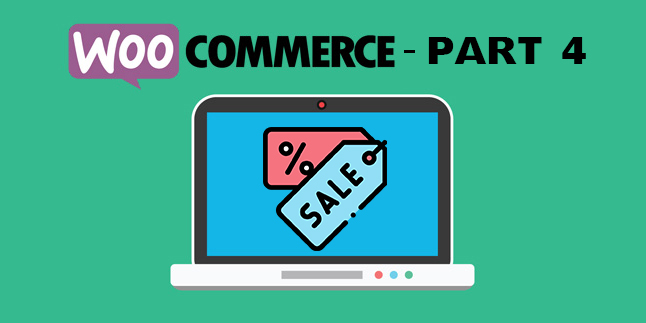
WooCommerce Part 4 – How to Hide the Coupon Field for a Specific Product Category
Hey hey—we’re back at it once again with our extremely popular WooCommerce coding series, where we give you totally free never-before-seen code that helps accomplish different functions with WooCommerce!
This week, we’re going to show you how to implement a much-requested function for WooCommerce that deals with coupons…
Imagine this scenario—you’ve got a t-shirt store, and you want to hide the coupon field in the shopping cart—but only for specific categories. How do you do it?
Well, WooCommerce doesn’t include this feature by default, and from what we’ve seen searching around on the web, some people have it pretty close, but not quite right in terms of how to implement this via PHP.
So, we’re going to drop some coding knowledge on you—totally gratis (that means free, but sounds way fancier ?).
As we always do, we’ll drop the full code snippet at the beginning of the article with code comments, and then we’ll break it down and explain what each part of the code is doing so you can understand easier. We see a lot of coding blogs/sites where someone posts a big snippet of code, but doesn’t really explain what it’s doing—which doesn’t help anyone who’s frustrated trying to implement this!
Without further ado, here’s the full PHP code require to conditionally hide coupons in a WooCommerce cart based on the product category:
add_filter( 'woocommerce_coupons_enabled', 'hide_coupon_field_specific_category', 20, 1 ); function hide_coupon_field_specific_category( $enabled ) { // Only on front-end if( is_admin() ) return $enabled; // Set the category name, ID, or slug here that you want to conditionally exclude coupons from $categories = array('tshirts'); // This will hide the coupon field in the tshirt category $found = false; // Boolean for checking // Loop through cart items foreach ( WC()->cart->get_cart() as $cart_item ) { if ( has_term( $categories, 'product_cat', $cart_item['product_id'] ) ){ $found = true; break; // End cart checking loop } } if ( $found && is_cart() ) { $enabled = false; } return $enabled; }
Now that you’ve seen all the code, let’s take a look at what the individual pieces are doing…
The first line you see is this:
add_filter( 'woocommerce_coupons_enabled', 'hide_coupon_field_specific_category', 20, 1 );
That’s a simple filter for WooCommerce. A filter is a hook that allows a plugin (in this case WooCommerce) to modify data at runtime (as the site is being loaded, in other words—real time).
We’re calling the hook for woocommerce_coupons_enabled which turns on/off coupons in WooCommerce. Then, we’re applying this hook based on the custom function we’re creating called hide_coupon_field_specific_category.
The next section of code you see is this:
function hide_coupon_field_specific_category( $enabled ) { // Only on front-end if( is_admin() ) return $enabled;
This code checks to see if you’re logged in as an admin, and if so, always displays the coupon field. This is important because it helps with debugging and testing.
After that, you’ll see the following code:
// Set the category name, ID, or slug here that you want to conditionally exclude coupons from $categories = array('tshirts'); // This will hide the coupon field in the tshirts category $found = false; // Boolean for checking
This is where the magic begins to happen. In this section, we’re defining two variables—one for a specific category you want to exclude, and a Boolean (true/false) variable to assist in checking.
After that, you’ll see this code:
// Loop through cart items foreach ( WC()->cart->get_cart() as $cart_item ) { if ( has_term( $categories, 'product_cat', $cart_item['product_id'] ) ){ $found = true; break; // End cart checking loop } }
The above code loops through the cart (the WooCommerce cart is an array and must be traversed with a loop). At each position in the loop, it checks for the item in the cart to be in the category you want to exclude.
Then, finally the following code is executed:
if ( $found && is_cart() ) { $enabled = false; } return $enabled;
The above code checks to see if any items in the category you want to exclude are found in the cart, and if they are, then the coupon prompt is disabled. However, if no items in the excluded category are found in the cart, then the coupon prompt is enabled. And that’s it!
One other important thing to remember is that this code section must go in your functions.php file in your WordPress theme in order to work properly.
For reference again, here is a link to the WooCommerce API hook index, if you’re looking to reference it in order to add to or take away from this function:
https://docs.woocommerce.com/wc-apidocs/hook-docs.html
We hope that this code gets you on your way to managing your WooCommerce coupons!