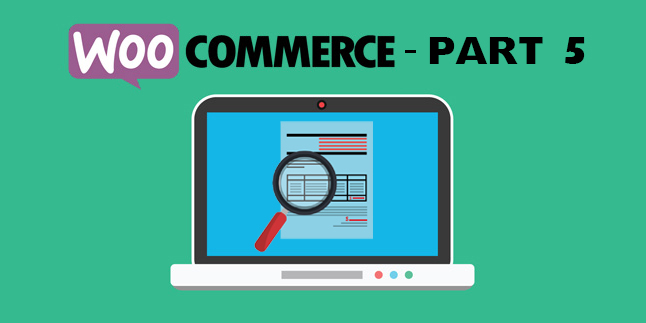
WooCommerce Part 5 – How to Exclude Products in a Specific Category from WooCommerce Search
Web Hosting Buddy here, back at it again with our WooCommerce coding series!
In today’s post, we’re going to cover something that seems to be a common question related to WooCommerce searching—how do you prevent products from a specific category from appearing in a search?
For example, let’s say that you’ve got an online clothing store that sells all different types of clothing (jeans, shoes, pants, suits, etc.), but for some reason, you don’t want any items from the t-shirt category to appear in the search results—what do you do?
Well, in this case, you’ll need a custom PHP function that adjusts the search query results just prior to runtime. And we’ve seen some other tutorials floating around the web on this topic, but the problem is that they don’t seem to work. Either the code is wrong, or it doesn’t work with the newest version of WooCommerce and WordPress.
Sound complicated? Well, it can be—but luckily we’ve written the code for you, so all you need to do is swap out our example category (t-shirts) with your own, paste this into your functions.php file, and you’re good to go!
Like always, we’ll show you the full code, and then look at each step to explain what’s happening under the hood.
Here’s the full code:
/* Remove Product Category from Search */ function remove_cat_from_search ( $q ) { if (!is_admin() && $q->is_main_query() && $q->is_search()) { $q->set( 'post_type', array( 'product' ) ); $tax_query = (array) $q->get( 'tax_query' ); $tax_query[] = array( 'taxonomy' => 'product_cat', 'field' => 'slug', 'terms' => array( 't-shirts' ), //no t-shirts in search 'operator' => 'NOT IN' ); $q->set( 'tax_query', $tax_query ); } } add_action( 'pre_get_posts', 'remove_cat_from_search' );
Now, let’s take a look at each step…
Here’s the first part of code we’re going to look at:
/* Remove Product Category from Search */ function remove_cat_from_search ( $q ) { if (!is_admin() && $q->is_main_query() && $q->is_search()) {
In this snippet, we add a comment to the function so we know what it does (in case we forget later on and go back to edit the code, etc.), and then we declare the function itself named “remove_cat_from_search.”
After that, we add an if statement that checks to see if the user is not an admin (no results are removed for admins), and then we check that it’s a main query, and then that the query is for a search. If all three of these things are true, then we proceed to the next steps.
Here’s the next snippet we’re looking at:
$q->set( 'post_type', array( 'product' ) ); $tax_query = (array) $q->get( 'tax_query' ); $tax_query[] = array( 'taxonomy' => 'product_cat', 'field' => 'slug', 'terms' => array( 't-shirts' ), //no t-shirts in search 'operator' => 'NOT IN' ); $q->set( 'tax_query', $tax_query ); } }
In this code, we’re setting/getting information about the query, and then using an array to modify that query so it does not contain an items from the t-shirts category. The “NOT IN” operator is used to exclude the taxonomy with a slug field containing “t-shirts” from the query. “NOT IN” is a SQL operator that allows us to perform this exclusion.
Then, after we’ve performed the exclusion, we set the new tax_query with the updated data and close off our functions with brackets that correspond to the if statement arguments and the entire function.
Note: when you use this code, don’t forget to change the “t-shirts” category to one of your categories that you want to exclude from search, or else it won’t work.
And here’s the last piece of code we’re going to look at:
add_action( 'pre_get_posts', 'remove_cat_from_search' );
This code hooks to our function on the “pre_get_posts” action, which fires after the query variable object is created, but most importantly—before the actual query is run. This allows for a seamless experience where users will never see any difference in the speed of the search, but the products in the category you want to hide will not be there.
And that’s it! We hope you’re able to apply this code to your site, and fine-tune your WooCommerce search for users!