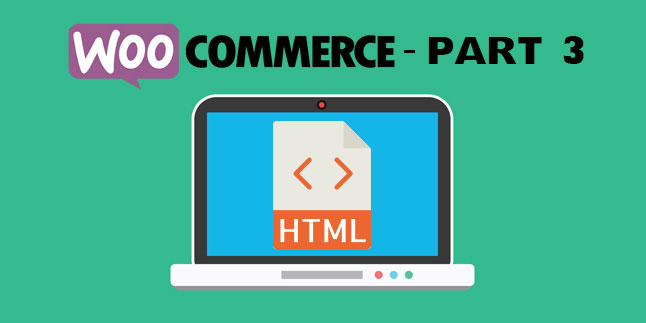
WooCommerce Part 3 – How to Open Specific Product Links in a New Tab or Window
Buckle up—it’s time for part 3 of the WooCommerce coding tips series! In this post, we’re going to look at how to open specific product links in a new tab or window when a user clicks on them—sounds simple, right?
The truth is that it’s actually pretty simple, it just requires a little bit of custom PHP coding to make it work with your WooCommerce installation. Let’s jump in and take a look at the problem!
The Problem: Opening Specific Product Links in a New Tab While Leaving Others to Open in the Current Window
Let’s take a look at an example! For instance, let’s say that you run a t-shirt store using WooCommerce, and you’ve got a variety of t-shirts.
Let’s also pretend that you’ve got a specific line of t-shirts that have dinosaurs on them, and for whatever reason, you want all of the single dinosaur t-shirt product pages to open in a new tab or window when clicked so that users don’t leave the main t-shirt store page to view the dinosaur t-shirts.
The Solution: A Custom PHP Function and a Little JQuery Magic
In order to do this, you’ll need to create some custom PHP combined with a little JQuery magic to select the div that the dinosaur t-shirt links are in.
Here’s the full custom function:
/* Add target="_blank" to WooCommerce Product Links on Specific Page */ add_action('wp_footer',function(){ if ( is_page( 'page-id-goes-here' ) ) { echo ''; } });
Let’s jump in an take a look at each part of the code to see what it’s doing:
/* Add target="_blank" to WooCommerce Product Links on Specific Page */ add_action('wp_footer',function(){
In this section, we’re simply calling a custom function in the footer to make sure that the entire page is loaded first (including all of the product links).
if ( is_page( 'page-id-goes-here' ) ) { echo '[BEGIN SCRIPT TAG GOES HERE]
Next, we’re telling PHP to check the page that the user is on in order to: 1) ensure it’s a page and 2) ensure it’s a specific page. WordPress uses numerical page IDs to refer to specific pages, and in this case, you simply replace ‘page-id-goes-here’ with your specific page ID, such as ‘543’ or whatever your page ID happens to be.
Side note: if you aren’t sure how to get the page ID it’s easy—just go into your WordPress admin panel, navigate to the pages section and hover your mouse over the page title (the link you click to edit the page). In the lower-left-hand corner of the screen, you’ll see the page ID displayed.
After checking the page ID, the function then calls a script (JQuery wrapped in the <script> tags) based on that conditional logic.
//select all links in product div jQuery("div.div-name-goes-here a").attr("target","_blank"); [END SCRIPT TAG GOES HERE]'; } });
Then, this JQuery checks the page for a specific div name and then applies a target_blank (open in new window/tab) attribute to that div.
For this to work properly, you have to wrap the product link that you want to open in a new tab/window in a div with a specific name. So, if you had a product called T-Rex Dinosaur Tee, you’d need to make sure that it’s wrapped in a div with an ID that can be referenced later, such as:
T Rex Dinosaur Tee Product PageThen, in the function listed above, you replace “div-name-goes-here” with the name of your t-rex div ID (t-rex-tee). So instead of the line “div.div-name-goes-here” you would have “div.t-rex-tee” etc.
And that’s it! After you follow these steps and clear your website cache at your hosting company etc., you should see your specific links opening in new tabs/windows.
Hopefully this helps anyone looking for a quick coding trick to open specific WooCommerce in a new tab/window! Stay tuned for part 4 of the WooCommerce coding series!
P.S. if you’re looking for the WooCommerce codex you can find that here: https://docs.woocommerce.com/documentation/plugins/woocommerce/woocommerce-codex/