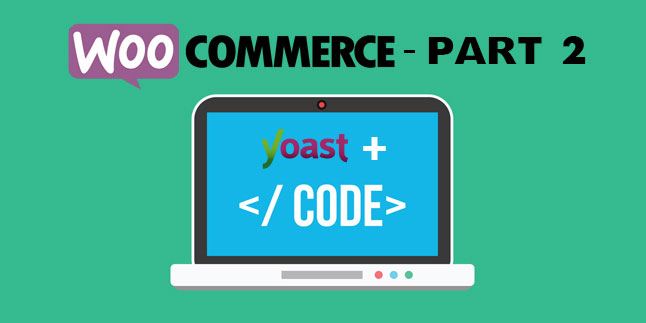
WooCommerce Part 2 – How to Noindex a Specific Category
We’re back again with another installment of our WooCommerce coding tips series! This time, we’re looking at a common problem that we couldn’t seem to find an existing solution for—how in the heck do you noindex all pages for a specific WooCommerce category?
This is actually quite easy if you’re using an SEO plugin—and for this example, we’ll look at Yoast (the most popular WordPress SEO plugin out there).
Understanding the Problem: Noindeinexing All Pages for a Certain WooCommerce Category
So, let’s say you have a category called “t-shirts,” and for whatever reason, you don’t want those pages to be indexed by search engines. An example reason for this could be there’s a section of your site that is duplicated for a specific purpose but contains the same merchandise such as fundraising t-shirts with special pricing vs. regular t-shirts (same products, but different pricing). Or, maybe there’s a product section you want live, but don’t want it to be accessible. Either way, noindexing the category might be a viable solution to avoid duplicate content and avoid user confusion.
The Solution: Creating a Custom PHP Function
In order to accomplish this, you’ll need a custom function where you add a Yoast filter function, and then use a WooCommerce category to run a conditional check. First, we’ll show you the code, and then (as always), we’ll walk through what each section is doing.
Here’s the Entire Function:
//noindex all products in specific category add_filter( 'wpseo_robots', 'yoast_seo_robots_remove_single' ); //add Yoast filter for meta function yoast_seo_robots_remove_single( $robots ) { if ( is_product() && has_term( 't-shirts', 'product_cat' ) ) { //check for t-shirt category return 'noindex,nofollow'; //noindex nofollow those pages } else { return $robots; //else return normal meta } }
Now, let’s take a look at what’s happening in each part:
//noindex all products in specific category add_filter( 'wpseo_robots', 'yoast_seo_robots_remove_single' ); //add Yoast filter for meta
In this first section of code, you’re creating a filter with Yoast to apply specific meta robots rules in the <head> section of your page. This is the section where noindex is set. Yoast typically pulls this from the settings section of the plugin, but in this case, we need to create a custom rule to override that for the category pages you don’t want to index.
function yoast_seo_robots_remove_single( $robots ) { if ( is_product() && has_term( 't-shirts', 'product_cat' ) ) { //check for t-shirt category return 'noindex,nofollow'; //noindex nofollow those pages
In the code above, we’re creating a custom function that actually removes the default Yoast settings based on a conditional if statement. What we’re saying is this—IF the page is a product page AND it is the t-shirts category, THEN apply a noindex/nofollow tag to the page.
} else { return $robots; //else return normal meta
Finally, in this last section of code, we’re saying this—IF the page is NOT a product page, AND also NOT t-shirts, then pull the normal meta settings plugin.
And, that’s it! When properly implemented, the following code will allow you to completely noindex specific WooCommerce categories and all pages that fall under that category.
If you’re looking for more information on WooCommerce functions and conditional operators, we recommend checking out their docs here:
https://docs.woocommerce.com/document/conditional-tags/
And if you need to reference the Yoast filter options, you can find those here:
https://yoast.com/wordpress/plugins/seo/api/
Hopefully now you’ve got everything you need to apply conditional indexing to your WooCommerce category pages!