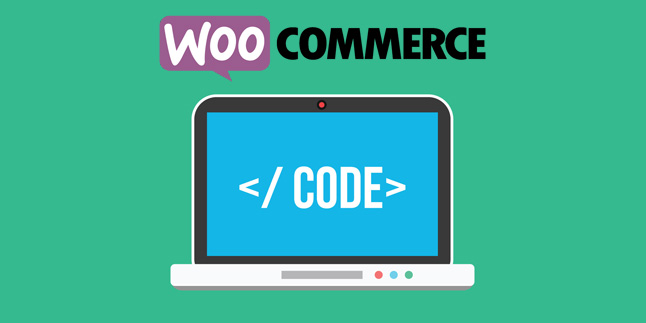
WooCommerce – Default Sort Method for a Specific Category
WooCommerce is an amazing WordPress plugin, but some of the default sorting options are not that robust. Sure, you can change the default sorting category, but this is applied globally and doesn’t help with category-specific sorting methods.
One of the biggest problems everyone has is this: what if you want to want to change the default sorting option, but only for a specific category or categories? Well, currently there’s no plugin to accomplish this, and no functionality in WordPress to do it either.
To add to the problem, there are tons of blogs where people post ways to make custom sorting options, remove sorting options, etc., but no one freaking explains how to have the default WooCommerce sorting method change based on the categories.
There are pages and pages of unanswered comments online on blogs everywhere, so—enter Web Hosting Buddy to the rescue! We whipped up some code to make this happen and it’s been tested/verified.
Here’s the code:
//custom function to override default sort by category function custom_default_catalog_orderby() { //choose categories where default sorting will be changed if (is_product_category( array( 'category1', 'category2', 'category3' ))) { return 'date'; // sort by latest }else{ return 'popularity'; // sort by popularity as the default } // end if statement } //end function add_filter( 'woocommerce_default_catalog_orderby', 'custom_default_catalog_orderby' ); //add the filter
In this example, we apply the “Sort by latest” sorting method (which is by most recent date) to category1, category2, and category3. Then, all other categories are sorted by popularity in the else statement.
Now, we’ll break it down and explain what the code is doing…
First, we create a custom function called custom_default_catalog_orderby.
//custom function to override default sort by category function custom_default_catalog_orderby() {
Next, we have a conditional if statement for WooCommerce to check the product categories. We used 3 example categories. This requires a PHP if conditional statement and an array for multiple categories.
//choose categories where default sorting will be changed if (is_product_category( array( 'category1', 'category2', 'category3' ))) {
Then, on these category pages we return the “Sort by latest” sorting method as default. WooCommerce calls this “date” in their PHP variables. If you’re modifying this, it’s important to note that variables used to call the sort methods aren’t the same as the drop-down GUI names.
return 'date'; // sort by latest
WooCommerce refers the GUI menu name “Sort by latest” as “date” in their PHP variables. If you’re modifying this and sorting by a different method, it’s important to note that the variables are as follows:
popularity = “Sort by popularity”
rating = “Sort by average rating”
date = “Sort by latest”
price = “Sort by price: low to high”
price-desc = “Sort by price: high to low”
With that said, let’s get back to the code…
Next, we have an else statement that returns popularity for all the other pages not checked in the above code (everything except category1, category2, and category3). This is important, because if you don’t have this section of code, you won’t be able to set a default sort for all of the other categories in WooCommerce settings because this code overrides everything.
}else{ return 'popularity'; // sort by popularity as the default } // end if statement } //end function
Finally, we have to add the filter to apply our code. Filters in WordPress allow hooks into plugins that modify data at runtime.
The filter that we’re calling is the “woocommerce_default_catalog_orderby” filter, which overrides the default order configured in the WooCommerce settings.
add_filter( 'woocommerce_default_catalog_orderby', 'custom_default_catalog_orderby' ); //add the filter
Now, what if you only want to apply a default sorting method to only one category instead of three? In that case, you’d remove the array and have a single category like this:
//choose categories where default sorting will be changed if (is_product_category( 'category1' )) {
And that’s it! If you’re looking to create a custom sorting method within the dropdown menu, the WooCommerce support docs have a great reference for that here:
https://docs.woocommerce.com/document/custom-sorting-options-ascdesc/
And, if you need to reference the WooCommerce conditional tags, you can find those here:
https://docs.woocommerce.com/document/conditional-tags/
Hopefully now you’ll be able to create some great customs sorting for your WooCommerce product pages/categories!