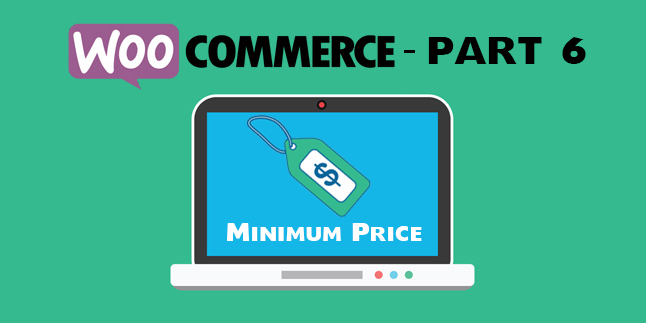
WooCommerce Part 6 – How to Display the Minimum Price on Product Category Pages
You know who it is—your coding pal, Web Hosting Buddy here, back at it again with the WooCommerce coding series!
In today’s installment, we’re going to touch on a popular WooCommerce coding question that doesn’t seem to have a good answer anywhere on the web, and that is—how do you display the minimum price for a product on product category pages, but show all the price variations on individual product pages?
To illustrate this concept, let’s go back to our t-shirt example. Suppose you’ve got an online clothing store and you sell a particular t-shirt that is more expensive depending upon the specific size.
So, for example, let’s say small and medium sizes are $10, large is $15, and XL or bigger is $20. But when someone is on the t-shirt product category page (yoursite.com/t-shirts/, etc.) you always want to show the lowest price for each item. How in the heck do you do that?
PHP to the Rescue – WooCommerce Custom Function for Minimum Price on Category Pages
In order to make this happen, we need to set the global price variable to the minimum price, and then revert this change when the page is an individual product page. So, let’s take a look at how this is done with PHP code.
In true Web Hosting Buddy fashion, we’ll provide you with the full code snippet, and then break down the parts piece-by-piece, so you understand exactly what the code is doing. Sound good? Alright, let’s jump in!
Here’s the full code snippet for this PHP function:
/* Display Minimum Product Price on Category Pages */ //Set the price variable as the minimum price everywhere add_filter( 'woocommerce_variable_sale_price_html', 'remove_variation_price', 10, 2 ); add_filter( 'woocommerce_variable_price_html', 'remove_variation_price', 10, 2 ); function remove_variation_price( $price , $product ) { $terms = get_the_terms( $product->id, 'product_cat' ); $price = $price; if ( $terms[0]->term_id == 50 /*replace 50 with your category ID*/ || $terms[0]->slug == 't-shirts' /*replace t-shirts with your category name*/ || $terms[0]->term_id == 100 /*replace 100 with your category ID*/ || $terms[0]->slug == 'jeans' /*replace jeans with your category name or delete the second statement if you only have one category you want to modify*/) { $variation_min_price = $product->get_variation_price('min'); $price = "$" . $variation_min_price; return $price; }else{ return $price; } } /* Remove Minimum Product Price on Product Pages */ //Now that we've set the product price as the minimum all the time in the function above, we need to revert this on product pages add_filter( 'woocommerce_variable_sale_price_html', 'remove_variation_price2', 10, 2 ); add_filter( 'woocommerce_variable_price_html', 'remove_variation_price2', 10, 2 ); function remove_variation_price2( $price , $product ) { $terms = get_the_terms( $product->id, 'product_cat' ); $price = $price; if ( is_product() && $terms[0]->term_id == 50 /*replace 50 with your category ID*/ || is_product() && $terms[0]->slug == 't-shirts' /*replace t-shirts with your category name*/ || is_product() && $terms[0]->term_id == 146 /*replace 50 with your category ID*/ || is_product() && $terms[0]->slug == 'jeans' /*replace t-shirts with your category name or delete the second statement if you only have one category you want to modify*/) { $price = ''; return $price; }else{ return $price; } }
So, let’s take a look at the first part of the code and see what’s going on here…
/* Display Minimum Product Price on Category Pages */ //Set the price variable as the minimum price everywhere add_filter( 'woocommerce_variable_sale_price_html', 'remove_variation_price', 10, 2 ); add_filter( 'woocommerce_variable_price_html', 'remove_variation_price', 10, 2 ); function remove_variation_price( $price , $product ) { $terms = get_the_terms( $product->id, 'product_cat' ); $price = $price;
In this section, we’re using the WooCommerce hooks “woocommerce_variable_sale_price_html” and “woocommerce_variable_price_html” to edit the displayed price, and then using adding a WordPress filter for our function “remove_variation_price.” Generally speaking, this code should be pasted into your functions.php file in WordPress.
In the next part of the code, we’re getting the WooCommerce product ID, and the product category in the loop, and then setting those as the $terms variable, and then setting our local variable $price as the price.
Now, let’s take a look at the next partial code snippet:
if ( $terms[0]->term_id == 50 /*replace 50 with your category ID*/ || $terms[0]->slug == 't-shirts' /*replace t-shirts with your category name*/ || $terms[0]->term_id == 100 /*replace 100 with your category ID*/ || $terms[0]->slug == 'jeans' /*replace jeans with your category name or delete the second statement if you only have one category you want to modify*/) { $variation_min_price = $product->get_variation_price('min'); $price = "$" . $variation_min_price; return $price; }else{ return $price; } }
In the above partial snippet, we’re testing some conditions with an IF statement. We’re saying that IF a product category equals a specific ID, OR, IF a product category name is t-shirts or jeans, THEN set the minimum price as the lowest variation and remove all other variations. Then to close our logic, we’re saying that IF none of the above is true (represented by the ELSE), THEN return the normal price variable.
Essentially, in simpler terms, we’re just telling WordPress to check to see if the product has a minimum price, and if it does, then set that price as the only product price.
But then there’s a problem that we have to account for—what happens when you’re on pages other than the product categories? Well, if we don’t add any additional code, then all pages will only display the lowest price and no variations, which is a problem.
So, to fix that problem, we add the following code:
/* Remove Minimum Product Price on Product Pages */ //Now that we've set the product price as the minimum all the time in the function above, we need to revert this on product pages add_filter( 'woocommerce_variable_sale_price_html', 'remove_variation_price2', 10, 2 ); add_filter( 'woocommerce_variable_price_html', 'remove_variation_price2', 10, 2 ); function remove_variation_price2( $price , $product ) { $terms = get_the_terms( $product->id, 'product_cat' ); $price = $price; if ( is_product() && $terms[0]->term_id == 50 /*replace 50 with your category ID*/ || is_product() && $terms[0]->slug == 't-shirts' /*replace t-shirts with your category name*/ || is_product() && $terms[0]->term_id == 146 /*replace 50 with your category ID*/ || is_product() && $terms[0]->slug == 'jeans' /*replace t-shirts with your category name or delete the second statement if you only have one category you want to modify*/) { $price = ''; return $price; }else{ return $price; } }
What we’re doing here is basically running the same code again (in an additional function and filters), and telling WordPress to test whether or not the current page is a product page, and if it is, then put the prices back to normal with the variations. And that’s it!
One important thing to note about this example is that you’ll need to get the product category IDs so you can plug them in the code, and you can get these by going on the categories page of your WordPress admin panel and hovering over the category name. If you look in the URL preview at the bottom of your browser, you should see the product category ID in the URL string.
Lastly, this example has been set up for more than one category in the IF statement. If you need two or more categories, you can just add conditions to the IF statement, and if you don’t, you can remove the second condition in this example (jeans) and just test for a single condition. Also, don’t forget to replace the example product category IDs (50 and 100) with your own, and replace the product category names (t-shirts and jeans) with your own.
And that wraps up part 6 of our WooCommerce coding series. Stay on the lookout for more great tips and tricks from Web Hosting Buddy!